Useful MongoDB Commands
I had the hardest time finding examples of how to connect to MongoDB via the command line and via a connection string in code. So I've compiled some helpful MongoDB commands here. For creating a database, creating a user, and connecting to the database.
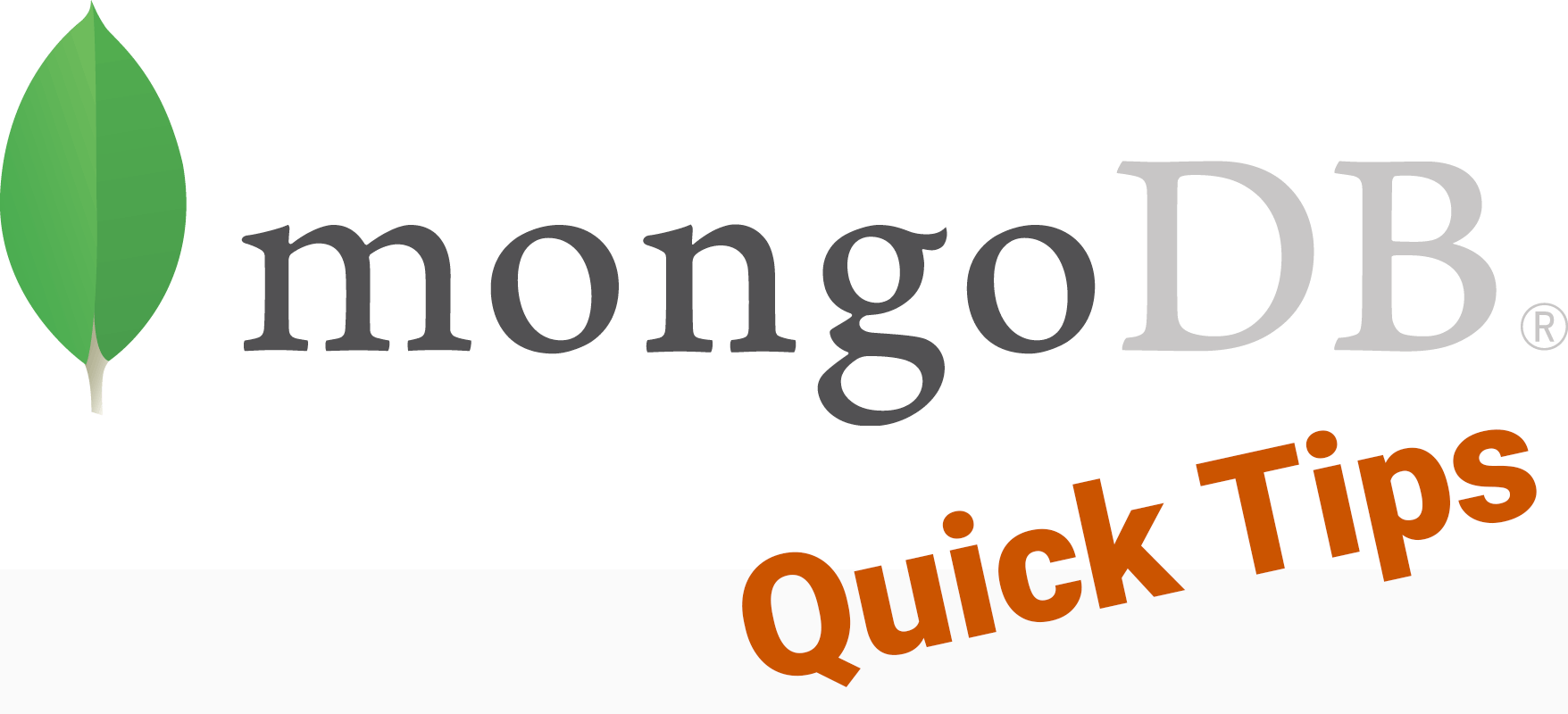
So you have a mongoDB server up and running? Nice work, now here are some useful mongodb commands:
I had the hardest time finding examples of how to connect to MongoDB via the command line and via a connection string in code so here is an example of both.
Connect to MongoDB From the command line:
./mongo -u testdbuser -p S3cur3P@ss --authenticationDatabase test mongodbserver/test
Here I'm calling the mongo command from inside a directory where I downloaded MongoDB.
-u - Flag to specify your MongoDB user
testdbuser - Your MongoDB username
-p - Flag to specify your MongoDB user's password
S3cur3P@ss - Your MongoDB user's password
--authenticationDatabase - Flag to specify the database to authenticate against
test - The database to authenticate against
mongodbserver - Your MongoDB server name or IP address
/ - To separate the MongoDB server name/IP from the database name
test - Your MongoDB database name
Connect to MongoDB From URL String:
mongodb://testdbuser:S3cur3P@ss@mongodbserver:27017/test
mongodb:// - Right
testdbuser - Your mongodb username
: - Separates the username and password
S3cur3P@ss - Your mongodb user's password
@ - Separates the credentials from the server information
mongodbserver - Your mongodb server name or IP address
: - Separates the server name/address from the port
27017 - The port to connect to your MongoDB server. The default port for MongoDB is 27017.
/ - Separates the database server information from the database name
test - Your MongoDB database name
To create a database and to switch to a database is the same command. If the database doesn't exist it will be created.
use test
Now that we are using a database lets create a user with read/write access.
db.createUser({
user: "testdbuser",
pwd: "S3cur3P@ss",
roles:[{
role: "readWrite",
db: "test"
}]
})
And here is how to change a user's password
db.changeUserPassword("testdbuser", "NEWS3cur3P@ss")
And here is how to add additional roles to a user
db.grantRolesToUser('testdbuser', [{
role: "readWrite",
db: "testdb2"
},{
role: "read",
db: "otherdb3"
},{
role: "readWrite",
db: "anotherdb5"
}])
And here is how to remove roles from a user
db.revokeRolesFromUser('testdbuser', [{
role: "readWrite",
db: "anotherdb5"
}])
Delete a user
db.dropUser('testdbuser')
To list all collections in the collection named mycollection
db.mycollection.find({})
To import a CSV file into a collection
$ mongoimport -h yourmongo.databaseserver.com -d mydb -c things --type csv --file locations.csv --headerline --authenticationDatabase admin --username 'iamauser' --password 'pwd123'
mongoimport - the command
-h - Flag to specify the database server hostname or IP address
yourmongo.databaseserver.com - Your database server name or IP
-d - Flag to specify the name of your database
mydb - Your MongoDB database name
-c - Flag to specify the collection to import the data into
things - The collection name we are importing into
--type - Flag to specify the type of file being imported
csv - The type of file we are importing
--file - Flag to specify the path to the file we are going to import
locations.csv - File name with absolute path to file
--headerline - Flag to specify the file's first row will be used for keys
--authenticationDatabase - Flag to specify the database to authenticate against
admin - Your MongoDB authentication database
--username - Flag to specify your MongoDB username
'iamauser' - Your MongoDB
--password - Flag to specify your MongoDB password
'pwd123' - Your MongoDB password
Drop/Delete a database
WARNING: THIS IS GOING TO DELETE THE DATABASE NAMED databaseName AND ALL CONTENTS WILL BE LOST!!!
use databaseName
db.runCommand( { dropDatabase: 1 } )